State Management in Flutter
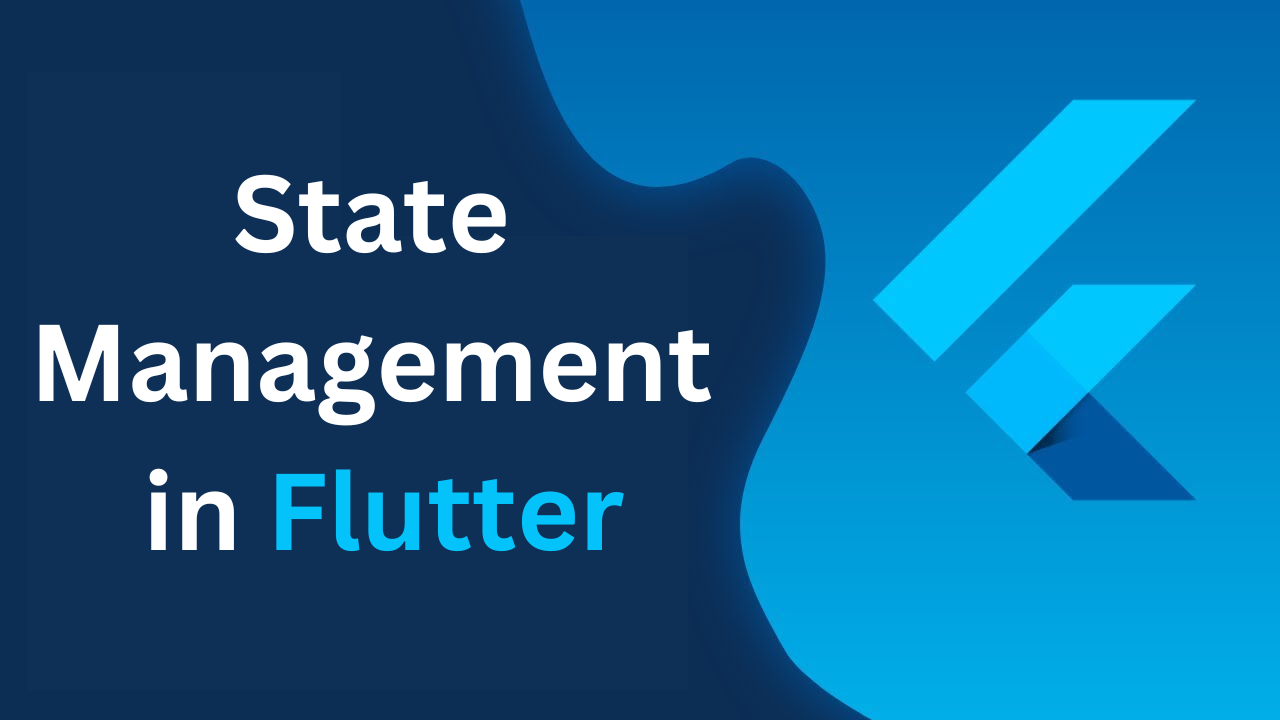
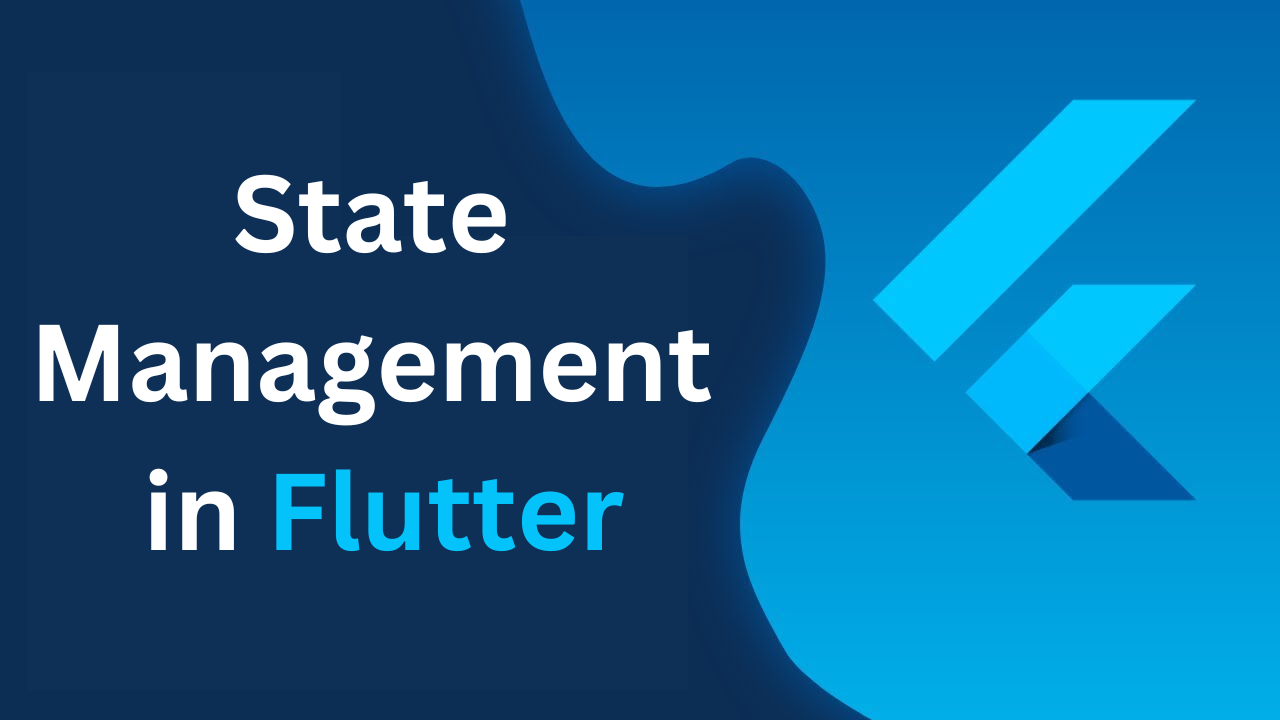
R. Vinoth
3 months ago
What is State Management?
Flutter is an innovative framework technology to build and deploy mobile and web apps on different platforms. Organising and controlling the state of these apps is a crucial part of Flutter called State Management. It can be used to control the behaviour appearance of the application UI. State management is how we manage and organise our app to most effectively access the state and share it across widgets.
Role of State Management
It provides access to the data.
Tell widgets that they need to be rebuilt when the data changes.
What is State in Flutter?
Before moving into the details of State Management, let us learn and understand what a state is. In Flutter, a state simply refers to the data that can change during the execution of the application. This means that there will be a change in the user interface along with the state change.
For example, Consider the case of a checkbox in the `terms and conditions screen’ of an application. Here when the user clicks on the checkbox, there will be a tick displayed in it but there won’t be any change in the other part of the screen. Thus only the state of the checkbox is changed.
Types of State
Ephemeral State: It refers to the type state in which data can be shared across only a single widget.
App State: It refers to the type state in which data can be shared across multiple widget.
Importance of State Management Libraries
State Management helps in creating a responsive user interface by controlling, managing and updating the UI efficiently. It results in creating well-structured and reusable code. The performance of an application must be maintained well by avoiding unwanted UI rebuilds, And proper state management helps in achieving this.
Performance
Code management
Code Reusability
Best State Management Libraries
Flutter offers a wide variety of State Management Libraries that are useful for managing app data according to the needs of your application. Some of the most commonly used State Management techniques are as follows,
Provider
Bloc
GetX
Riverpod
1) Provider
Provider is the most recommendable state management package for beginners and freshers. The basic objective of a Provider object is to store the data and transfer it to another widget that needs it. Most of the basic flutter projects can be efficiently achieved through state management using the provider. To accomplish state management with the provider, we need to use the ‘provider’ package.
Adding Package
To get started, We need to add the provider package from Pub. dev site to the ‘pubspec.yaml’ file of our flutter project,
dependencies:
flutter:
sdk: flutter provider: ^6.1.1
To ensure the package version is correctly installed, we need to run 'flutter pub get'
Import Library
To use the installed provider, we need to import this library
import 'package:provider/provider.dart';
Advantages of Provider
Mainly adaptable and easy to understand for freshers.
It can also be used with any architecture as per the user's demands.
2) Bloc
Bloc is an acronym for Business Logical Components. It is a powerful package and also one of the most commonly used State Management Library. It is suitable for managing and controlling data states of larger applications. As the name indicates, it helps in filtering out business logic from the user interface logic.
Using Bloc helps to improve the performance and easy to reuse the code.
Adding Package
In the case of Bloc, we are using the package called ‘flutter_bloc’ in our project
dependencies:
flutter:
sdk: flutter flutter_bloc: ^8.1.3
To ensure the package version is correctly installed, we need to run ‘flutter pubget’
Import Library
import ‘package:flutter_bloc/flutter_bloc.dart';
Advantages of Bloc
Bloc helps to separate business logic from user interface logic and allows the user to manage data more efficiently.
Bloc provides importance to scalability, reusability and code organisation to your application.
3) GetX
GetX is another important package that is widely used in Flutter for State Management for developing efficient applications. GetX does not use Streams and ChangeNotifiers like we use in Provider and Bloc. Navigating to other widgets is easier in GetX while comparing with other State Management Packages such as Provider, Bloc..etc. Dependency injection and Route management are also some of the efficient features of the GetX package.
Adding Package
From the pub.dev site we add the ‘get’ package to our pubspec.yaml file
dependencies:
flutter:
sdk: flutter get: ^4.6.6
To ensure the package version is correctly installed, we need to run ‘flutter pubget’
Import Library
To access the features of GetX, we need to import this package to your code
import 'package:get/get.dart';
Advantages of GetX
GetX allows us to manage dependencies much more simply through dependency injection features and helps to build scalable and stable apps.
In GetX, Navigation to other pages is done easily and efficiently using Get.to( NextScreen () );
4) Riverpod
Riverpod is also a sophisticated and important Flutter state management pack that allows you to manage complex states and dependencies. Riverpod is introduced to overcome the limitations of the Provider package. It helps in disposing
state of providers that are unwanted. Retrieving and caching data is well organisedin Riverpod which helps you to build an attractive and efficient application.
Adding Package
To begin with Riverpod, we need to import the ‘flutter_riverpod’ package from pub. dev to our pubspec.yaml file.
dependencies:
flutter:
sdk: flutter flutter_riverpod: ^2.4.9
To ensure the package version is correctly installed, we need to run ‘flutter pubget’
Import Library
Now in your dart code, paste this into the header
import ‘flutter_riverpod/flutter_riverpod.dart’;
Advantages of Riverpod
Since Riverpod is a solution to the barriers of the Provider package, it helps in creating providers of the same type in a project which was not possible with Provider.
We can define global providers using Riverpod.
Conclusion
In conclusion, we learned about State Management and various State Management Libraries used in Flutter such as Provider, Bloc, GetX and Riverpod. As a Flutter developer, it is an important factor to choose the appropriate state management based on the nature and need of your application. Building multi-platform apps using Flutter is fun and choosing the proper state management helps you to create attractive and interesting applications that work effectively. Code Your Best!